React + Spring Boot for Beginners: A Step-by-Step Roadmap to a Solid Full Stack

Mandar J
Last updated:
When you're building a modern web application, you need to think about both the front-end and the back-end. The front-end is what users interact with—the buttons they click, the forms they fill out, and the pages they view. The back-end is what powers the application behind the scenes—it processes data, stores information, and makes everything work smoothly.
Why React + Spring Boot?
React is a powerful front-end library developed by Facebook. It allows developers to build dynamic, user-friendly interfaces. It's all about creating components—small, reusable pieces of code that you can use to build bigger, more complex interfaces.
Spring Boot, on the other hand, is a back-end framework built on Java's Spring framework. It simplifies the process of creating stand-alone, production-ready applications. It helps you create robust, scalable, and maintainable back-end systems that handle all the heavy lifting.
By combining React with Spring Boot, you get the best of both worlds—a slick, responsive front-end paired with a powerful, efficient back-end. This combination is ideal for building full-stack applications that are both user-friendly and capable of handling complex business logic.
RoadMap:
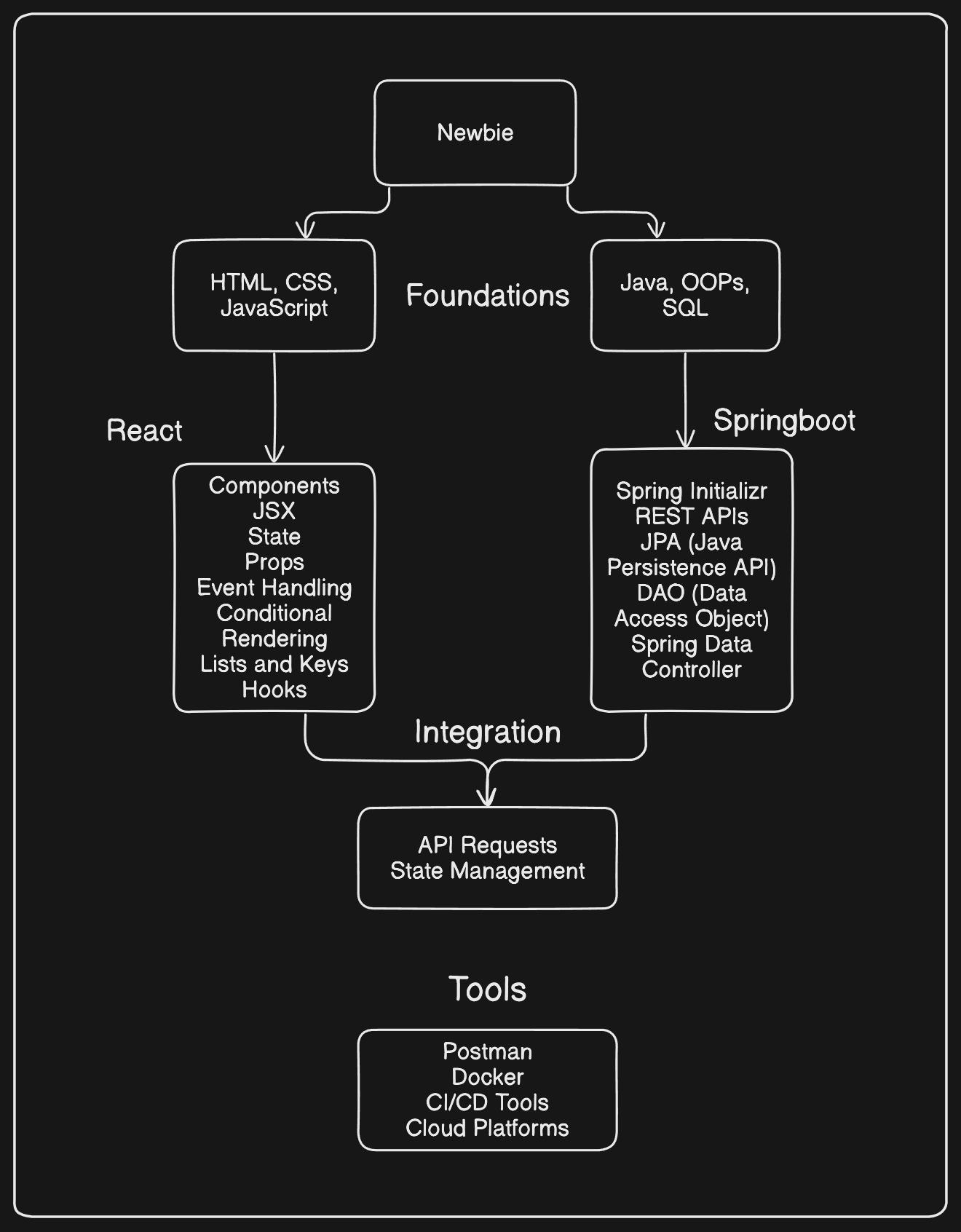
Foundations: HTML, CSS, JavaScript, Java
Before diving into React and Spring Boot, it's essential to understand the foundations:
HTML (HyperText Markup Language): HTML is the skeleton of your web pages. It structures content, such as text, images, and links, so that browsers can display them correctly. Think of it as the blueprint of a house—it outlines what goes where.
CSS (Cascading Style Sheets): While HTML is the structure, CSS is the style. It controls the look and feel of your web pages—colors, fonts, layout, and more. If HTML is the blueprint, CSS is the interior design. It makes everything look good and feel right.
JavaScript: JavaScript is the programming language of the web. It makes your web pages interactive. When you see a button that changes color when you hover over it, or a form that checks for errors before submission, that’s JavaScript in action. It's what brings your website to life. Checkout: 10 JavaScript Concepts to Revisit Pre-React.
Java: Java is a versatile programming language used to build everything from mobile apps to large-scale enterprise systems. Core Java refers to the basic concepts of Java, including object-oriented programming, data types, loops, and more. It's the foundation of many powerful back-end technologies, including Spring Boot.
React Fundamentals
Once you're comfortable with JavaScript basics, focus on the core concepts of React(if not, checkout: 10 JavaScript Concepts to Revisit Pre-React).
Components: Learn about functional and class components. Understand how to create reusable components and pass data between them using props.
JSX: Get to know JSX, the syntax extension that looks like HTML but is actually JavaScript. Understand how JSX is compiled to JavaScript.
State and Props: Master state management within components and passing data through props.
Event Handling: Know how to handle user interactions, such as clicks and form submissions.
Lifecycle Methods: For functional components, understand the use of useEffect.
React Hooks
With the introduction of hooks, functional components have become more powerful. Get familiar with:
Basic Hooks: useState, useEffect, useContext, and how they are used to manage state and side effects.
Custom Hooks: Learn how to create your own hooks to encapsulate logic and reuse it across components.
Routing
For most applications, you'll need to manage navigation. Learn how to use React Router to handle routing and navigation within your app.
State Management
As your app grows, managing state becomes more complex. Understand the basics of state management libraries like Redux or the Context API, and how they help in managing global state.
Basic CSS/Styling
Know how to style your React components. This includes traditional CSS, CSS-in-JS solutions like styled-components, and CSS modules.
Working with APIs
Most applications need to fetch data from external sources. Understand how to make API requests using fetch or Axios, and handle the responses.
Version Control
Basic knowledge of Git is essential for version control. Learn how to commit changes, create branches, and collaborate with others.
Build Tools
Familiarize yourself with build tools like Webpack and Babel, as well as package managers like npm or yarn. These tools help manage dependencies and streamline the development process.
REST APIs, Web Services, and the Need for Back-End Microservices
When your front-end needs to communicate with your back-end, it often does so through REST APIs (Representational State Transfer Application Programming Interfaces). A REST API is a set of rules that allows different software applications to communicate with each other over the web.
Web Services:
These are services offered by one device to another through the web. They allow different applications to talk to each other. For instance, when you use a weather app on your phone, it likely communicates with a web service to fetch the latest weather data.
Back-End Microservices:
Traditionally, back-end systems were built as monolithic applications—a single, large codebase that handled everything. While this approach works, it can become difficult to manage and scale as the application grows. This is where microservices come in.
Microservices break down the back-end into smaller, independent services. Each service handles a specific piece of functionality, like user authentication or payment processing. This makes the system more flexible and easier to maintain. If one service fails, it doesn’t bring down the entire application.
Spring Boot shines here because it makes it easy to build microservices. It comes with all the tools you need to create and deploy microservices quickly and efficiently.
Spring Boot Concepts
Spring Boot simplifies the process of building Java applications, but as you dive deeper, there are more powerful tools and concepts to explore. Let’s break down some of the intermediate concepts that can take your Spring Boot applications to the next level.
Spring and Spring Boot: Quick Recap
Spring Framework: A powerful toolkit for building Java applications, but it can be complex for beginners.
Spring Boot: A streamlined version of Spring that handles most of the setup for you, letting you focus on writing your application.
Spring Initializr: Starting Off Right
Spring Initializr helps you kickstart your Spring Boot project by providing a ready-made structure. It’s like having a project blueprint with all the essential tools you need to start building immediately.
HTTP Methods and REST APIs: Web Communication Made Simple
GET, POST, PUT, DELETE: These are basic commands (HTTP methods) that let your app communicate over the web through REST APIs. Spring Boot makes it easy to create these APIs with minimal code.
Data Access with JPA: Simplifying Database Interaction
JPA: A tool that makes it easy to store and retrieve data from your database without writing complex SQL queries.
Connecting to a Database: Spring Boot handles the heavy lifting, allowing you to connect to databases like MySQL or H2 with just a few configuration steps.
DAO Service: Keeping Your Code Tidy
DAO (Data Access Object): This design pattern keeps your database logic separate from your business logic, making your code cleaner and easier to manage.
Feign Client: Communicating with Other Services
Feign: A tool that simplifies communication between your application and other services (like other microservices). Instead of writing detailed HTTP client code, you define what you need, and Feign handles the rest.
Service Discovery: Finding Microservices Automatically
In a microservices architecture, your application may consist of many small services working together. Service Discovery helps these services find each other without hardcoding their locations.
Eureka: A popular tool used with Spring Boot for service discovery. It acts like a directory where services can register and discover other services automatically. For example, if your app’s user service needs to communicate with the payment service, Eureka helps them find each other even if their locations change.
Centralized Logging: Keeping Track of Everything
As your application grows, keeping track of what’s happening becomes critical. Centralized Logging is a way to collect logs from all parts of your application in one place.
Spring Boot Actuator: A built-in tool that provides insights into your application’s running state. It also integrates with logging frameworks to help you monitor performance, errors, and other important events.
Logback and Log4j: These are popular logging frameworks that work with Spring Boot. They allow you to capture detailed logs from different parts of your application, which can be stored in a central location for easy access and analysis.
Spring Security: Protecting Your Application
Security is essential for any application, and Spring Security offers powerful tools to protect your app.
Authentication and Authorization: Spring Security helps manage who can access your application and what they can do. For example, it can ensure that only logged-in users can access certain features or that only admins can perform specific actions.
Integration with OAuth2 and JWT: Spring Security can integrate with popular security protocols like OAuth2 (for authentication) and JWT (JSON Web Tokens) for secure communication between services.
Spring Cloud Config: Managing Configuration Across Services
When you have multiple microservices, managing their configurations can become a headache. Spring Cloud Config centralizes your configurations in one place, making it easy to update and manage settings across all your services.
Centralized Configuration: Instead of configuring each service individually, Spring Cloud Config allows you to manage all your configurations from a single location. This makes it easier to maintain consistency and quickly update settings across all your microservices.
Build a Simple Seat Reserving App with React + Spring Boot
1. Set Up Spring Boot Back-End
Create Project: Use Spring Initializr with Web, JPA, and H2 dependencies.
Define Entity: Create a Seat class with fields like blogId, number, and reserved.
Repository: Set up SeatRepository extending JpaRepository for CRUD operations.
Controller: Implement SeatController with REST API endpoints for getting available seats, reserving a seat, and canceling a reservation.
Test: Use Postman or curl to check API functionality.
2. Set Up React Front-End
Create Project: Use create-react-app to start a new React project.
Components: Develop SeatList, SeatItem, and ReservationForm components.
State Management: Use useState to manage seat data and useEffect to fetch data from the back-end.
Functionality: Implement functions to reserve and cancel seats via API calls.
Test: Run the React app locally to ensure proper functionality.
3. Connect Front-End and Back-End
Configure CORS: Allow requests from your React app in the Spring Boot application.
Deploy: Deploy React on Netlify/Vercel and Spring Boot on Heroku/AWS.
Verify: Test the full application in a production environment to ensure that seat reservations work correctly.
Conclusion:
In summary, this roadmap guides you from foundational concepts to advanced techniques in React and Spring Boot. By mastering HTML, CSS, JavaScript, Core Java, and SQL, and then diving into React and Spring Boot basics, you’ll build a solid full-stack development skill set. The hands-on experience with integrating front-end and back-end technologies, combined with intermediate tools and techniques, will empower you to create robust, dynamic web applications. Keep exploring and experimenting to advance your skills and build impactful projects.
Related Blogs
August 30, 2024
August 24, 2024
April 20, 2024
April 12, 2024